How to Design a Menu with Flutter
Learn how to design a menu using Flutter, a popular cross-platform framework for building beautiful and interactive mobile applications. Discover the techniques and best practices for creating an intuitive and visually appealing menu design in your Flutter projects.
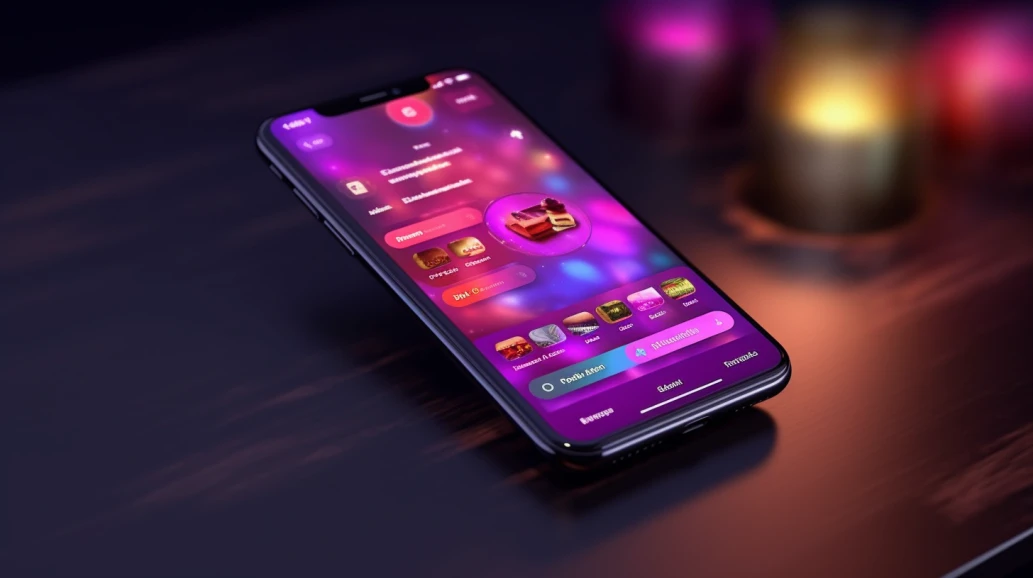
Flutter is a popular mobile app development framework that allows developers to build high-quality, performant apps for both Android and iOS platforms. One key aspect of any mobile app is its user interface, and designing an intuitive and attractive menu is an important part of creating a great user experience. In this article, we will explore how to design a menu with Flutter using the drawer package. We will start with a simple menu and gradually add customizations to make it more visually appealing and functional. By the end of this article, you will have the knowledge and tools to create a professional-looking menu for your Flutter app.
If despite these explanations you have difficulty designing your Flutter menu, register on Univext and book a lesson. Our teachers will be able to help you!
Getting Started
To get started, you need to have Flutter installed on your machine. Here are some steps from installing the app to creating your first Flutter project. However, if you want more details on installing Flutter, you can read our article: How to create your first simple mobile application with Flutter in under 15 minutes.
- Install Flutter: Before creating a new Flutter project and being able to design a menu, you need to have Flutter SDK installed on your machine. You can download the Flutter SDK from the official website: https://flutter.dev/docs/get-started/install.
- Set up your development environment: After installing Flutter, you need to set up your development environment. You can follow the instructions provided in the Flutter documentation to set up your development environment for your specific operating system: https://flutter.dev/docs/get-started/install.
- Open your IDE: Open your favorite IDE (Integrated Development Environment) that supports Flutter development, such as Android Studio, Visual Studio Code, or IntelliJ IDEA.
- Create a new Flutter project: In your IDE, select "Create New Flutter Project" from the welcome screen or the main menu.
- Configure your new project: In the new project wizard, choose the type of project you want to create, such as an App or a Package. You can also set the project name, package name, location, and other settings.
- Finish creating your new project: After configuring your new project, click "Finish" to create your new Flutter project. Your IDE will generate the necessary files and directories for your new project.
flutter create myapp
Congratulations! You have now created a new Flutter project. You can now start designing your menu.
Designing the Menu
Definition of Package
To design a menu with Flutter, we will use a package. In Flutter, a package is a pre-built library or module of code that developers can use to add new features or functionality to their app. Packages can be thought of as a collection of reusable code that can be shared across multiple projects. They provide a way for developers to quickly and easily add new features to their app without having to write all the code from scratch.
In this article, we use a package called drawer. The drawer package provides a widget called Drawer that allows us to create a side menu in our app. The Drawer widget is a pre-built implementation of a common UI pattern that is used in many mobile apps. By using the drawer package, we can save time and effort in implementing a menu in our app and instead focus on other parts of our app that require more custom code.
The drawer package is a part of the official Flutter SDK and is maintained by the Flutter team at Google. It is a popular and widely-used package in the Flutter community, making it a reliable and safe choice for adding a menu to our app.
Use of Package
To use the drawer package, we need to add it to our project's dependencies in the pubspec.yaml
file. Here's an example of how to do that:
dependencies:
flutter:
sdk: flutter
drawer: ^0.3.1
Once we have added the drawer package to our project, we can start building the menu. Here's an example of how to create a simple menu:
import 'package:flutter/material.dart';
import 'package:drawer/drawer.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'My App'
home: Scaffold(
appBar: AppBar(
title: Text('My App'),
),
drawer: Drawer(
child: ListView(
padding: EdgeInsets.zero,
children: <Widget>[
DrawerHeader(
child: Text('My App'),
decoration: BoxDecoration(
color: Colors.blue,
),
),
ListTile(
title: Text('Option 1'),
onTap: () {
// TODO: Handle option 1 tap
},
),
ListTile(
title: Text('Option 2'),
onTap: () {
// TODO: Handle option 2 tap
},
),
],
),
),
body: Center(
child: Text('Main Content'),
),
),
);
}
}
Let's break down this code and see what's happening. We start by importing the drawer package and creating a new Flutter app with MyApp
as the root widget. We then create a
Scaffold
widget, which is a container for our app's UI elements. We add an AppBar
to the Scaffold
widget to display a title for our app. We then add a Drawer
widget
to the Scaffold
widget by setting the drawer
property. The Drawer
widget contains a ListView
widget that lists the navigation options.
We add a DrawerHeader
widget to the ListView
widget to display a header for our menu. We set the child
of the DrawerHeader
widget to a
Text
widget with the title of our app. We also set the decoration
property of the DrawerHeader
widget to set a background color.
We add two ListTile
widgets to the ListView
widget to represent the navigation options. We set the title
property of each ListTile
widget to the
name of the navigation option. We also set the onTap
property of each ListTile
widget to update the UI when the user taps on the option. In this example, we just leave
the onTap
property empty.
Finally, we add a Center
widget to the Scaffold
widget to display the main content of our app.
That's it! We now have a simple menu in our app that users can access by swiping from the left side of the screen.
Customizing the Menu
The drawer package provides several properties that we can use to customize the appearance and behavior of the menu. Let's take a look at some of these properties:
Header
We can customize the header of the menu by setting the header
property of the Drawer
widget. This property allows us to set a custom widget as the header of the menu. Here's
an example:
Drawer(
header: Text('My Custom Header'),
child: ...,
)
Footer
We can customize the footer of the menu by setting the footer
property of the Drawer
widget. This property allows us to set a custom widget as the footer of the menu. Here's
an example:
Drawer(
header: Text('My Custom Footer'),
child: ...,
)
Background Color
We can set the background color of the menu by setting the backgroundColor
property of the Drawer
widget. Here's an example:
Drawer(
backgroundColor: Colors.blue,
child: ...
)
Item Color
We can set the text color of the menu items by setting the itemColor
property of the ListView
widget. Here's an example:
Drawer(
child: ListView(
itemColor: Colors.red,
children: ...
),
)
Item Icon
We can set an icon for each menu item by setting the leading
property of the ListTile
widget. Here's an example:
ListView(
children: <Widget>[
ListTile(
leading: Icon(Icons.home),
onTap: () {
// Update the UI
},
),
Item Badge
We can add a badge to each menu item by setting the trailing
property of the ListTile
widget. Here's an example:
ListView(
children: <Widget>[
ListTile(
title: Text('Option 1'),
trailing: Chip(
label: Text ('3')
)
onTape: () {
// Update the UI
},
);
Conclusion
Designing a menu is an important part of creating a great user experience in any mobile app. With Flutter, we can use the drawer package to quickly and easily create a side menu in our app. By starting with a simple implementation and gradually adding customizations, we can create a professional-looking and functional menu that meets the specific needs of our app and our users. The drawer package is a reliable and widely-used package in the Flutter community, and by using it, we can save time and effort in implementing a menu in our app. With the knowledge and tools provided in this article, you can confidently design a menu with Flutter for your next mobile app project.
If you have difficulties and you do not understand certain concepts or procedures, our teachers are waiting for you on Univext. They are there to help and support you! Register on Univext and book your first Flutter lesson!